In this blog post, we’ll explore a simple and practical PowerShell script that helps you list files in a directory along with their sizes and creation dates. This script provides a straightforward way to gain insights into your file system, making it easier to manage files based on both their size and when they were created.
List Files with Size and Last Write Time
This PowerShell script below provides administrators with a quick overview of the files in the current directory, ordered by file size (MB) from highest to lowest.
# PowerShell Script: List Files with Size and Last Write Time $files = Get-ChildItem -File $fileList = $files | Select-Object Name, LastWriteTime, @{Name="SizeMB"; Expression={[math]::Round($_.Length / 1MB, 2)}} $sortedFileList = $fileList | Sort-Object -Property SizeMB -Descending $sortedFileList | Format-Table -AutoSize
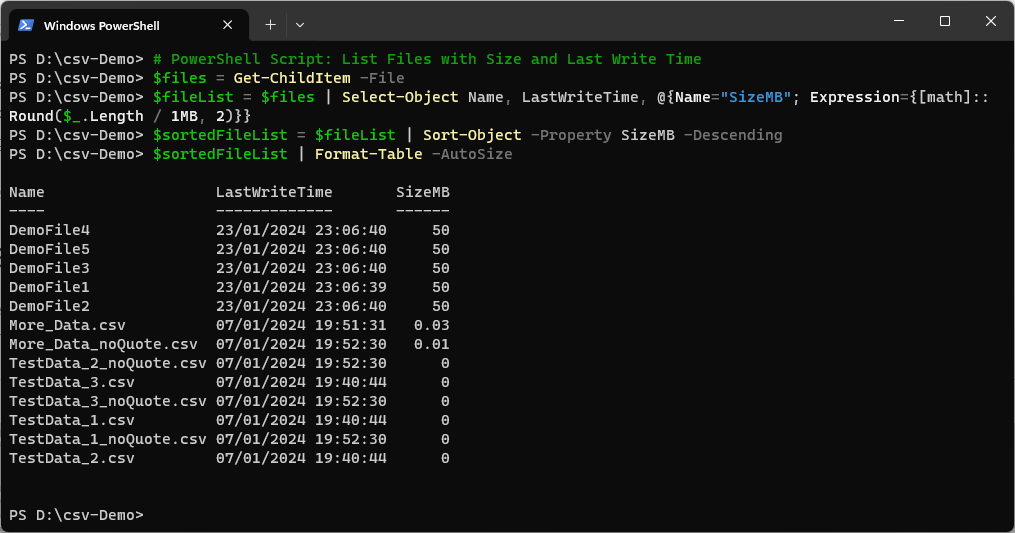
How It Works
Get-ChildItem: Retrieves all files in the current directory using the -File
parameter.
Select-Object: Creates a custom object for each file with its name, last write time, and size in megabytes.
Sort-Object: Orders the files by size in descending order.
Format-Table: Displays the sorted file list in a table format.
Practical Application (Make it a Function)
Understanding how to list files with both sizes and creation dates is valuable for various scenarios:
– File Organization: Quickly identify and organize files based on their sizes and when they were created.
– Storage Management: Easily locate large or old files that might be candidates for archiving or deletion.
– Audit Trail: Keep track of file creation dates for auditing purposes.
All of the above is made easier if we make the code into a function!
# PowerShell Function: List-FilesWithSizesAndDates Function List-FilesWithSizesAndDates { param( [string]$directoryPath = (Get-Location) ) $files = Get-ChildItem -Path $directoryPath -File $fileList = $files | Select-Object Name, CreationTime, @{Name="SizeMB"; Expression={[math]::Round($_.Length / 1MB, 2)}} $sortedFileList = $fileList | Sort-Object -Property SizeMB -Descending $sortedFileList | Format-Table -AutoSize } # Example usage: List files in the current directory List-FilesWithSizesAndDates # Example usage: List files in a specific directory # List-FilesWithSizesAndDates -directoryPath "d:\mssql_backups"
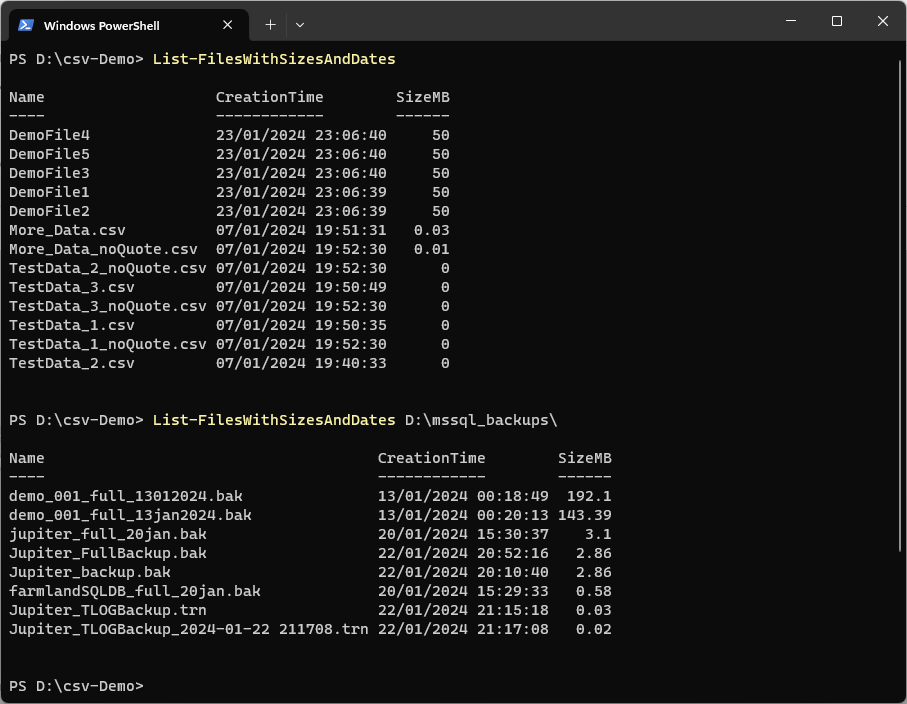
This script has been transformed into a function named List-FilesWithSizesAndDates
. The function takes an optional parameter $directoryPath
to specify the directory to list files from. If no path is provided, it defaults to the current directory.
To use the function, simply call List-FilesWithSizesAndDates
without any parameters to list files in the current directory. Alternatively, you can specify a directory path as shown in the example usage comment.
Hope this is useful for you!
Leave a Reply