Today we are going to explore the concept of PowerShell ‘Tail,’ a technique inspired by the Unix ‘tail’ command, to efficiently monitor and analyze log files in real-time.
Whether you’re a system administrator, developer, or IT professional, mastering PowerShell cmdlets such as Get-Content, can significantly enhance your log analysis capabilities. In this post, we will get the last rows of a log file with the Get-Content
cmdlet, and then create a PowerShell function that makes it easier going forward.
Getting Last Lines of Log File PowerShell
The PowerShell command below will return the last 10 lines of a .txt log file using the Get-Content PowerShell cmdlet:
# Return last lines of log file Get-Content .\appLog0001-0500.txt | Select-Object -Last 10
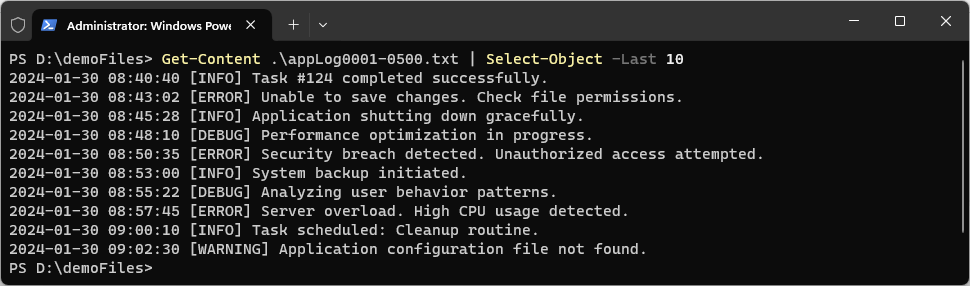
This simple yet effective command is invaluable for troubleshooting an issue or real-time monitoring for diagnostics.
Create a PowerShell Tail-Log Function
The PowerShell script below creates a function named Tail-Log
that facilitates the analysis of log files.
This PS function takes a log file path as a mandatory parameter and an optional parameter for the number of lines to display, with a default of 10. It begins by checking the existence of the specified log file and raises an error if it does not exist. Subsequently, it reads the last N lines from the log file using the Get-Content
cmdlet. The function then iterates through each line, applying distinct text colors based on predefined keywords such as “Critical,” “Important,” and “Warning.”
To implement this function in your PowerShell terminal, run the script provided:
# Function definition for Tail-Log function Tail-Log { param( [string]$LogFile, [int]$LinesToDisplay = 10 ) # Check if the log file exists if (-not (Test-Path $LogFile -PathType Leaf)) { Write-Error "Log file not found: $LogFile" return } # Read the last N lines from the log file $logContent = Get-Content $LogFile -Tail $LinesToDisplay # Output each line with appropriate colors foreach ($line in $logContent) { if ($line -match 'critical') { Write-Host $line -ForegroundColor DarkRed } elseif ($line -match 'important') { Write-Host $line -ForegroundColor DarkYellow } elseif ($line -match 'warning') { Write-Host $line -ForegroundColor Yellow } else { Write-Host $line } } }
Example usage of Tail-Log function
This PowerShell function we have created has an optional parameter for specifying the number of lines to display (default is 10).
# Return last 10 lines of a log file Tail-Log -path "C:\temp\logfile001.txt" 10
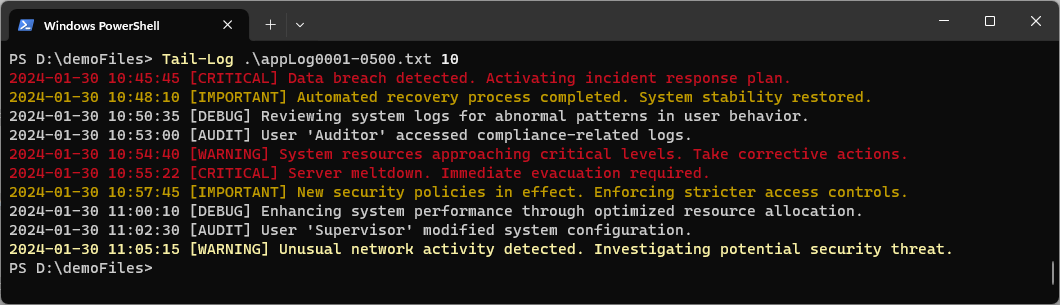
In this output example, one of the [WARNING] lines shows as dark red, this is because it line also includes the word ‘Critical’.
Practical Use Cases of Tail-Log:
Troubleshooting System Events:
– Use PowerShell Tail-Log for swift monitoring of system logs, aiding in the quick identification of potential issues and efficient troubleshooting.
– Troubleshoot script executions by accessing the last lines of relevant logs, enabling rapid error response and resolution.
– Enhance security auditing with Tail-Log for real-time monitoring and analysis of security-related logs, ensuring timely detection and response to potential threats.
Monitoring Application Logs:
– Tail logs for critical applications, providing instant error detection and proactive maintenance to uphold application health.
– Monitor application logs for performance issues or anomalies, facilitating prompt interventions to optimize application functionality and enhance user experience.
Customization for Admins:
– Tailor the Tail-Log script to specific needs, allowing administrators to customize log filtering based on keywords or adjust color-coded outputs.
– Refer to the Microsoft Docs page on Console.ForegroundColor (Microsoft Docs) for a comprehensive guide to color choices, enhancing the visual representation of log entries.
– Experiment with output formatting options, such as timestamps or log entry types, to enhance the visual representation according to specific preferences and requirements.
Automation with Scheduled Tasks:
– Streamline log monitoring by integrating PowerShell Tail with scheduled tasks, automating script execution at specified intervals.
Conclusion
In conclusion, this blog post serves as a comprehensive and accessible guide, effectively communicating the advantages and applications of the PowerShell Tail concept. The inclusion of practical examples, usage scenarios, and customization tips transforms it into a valuable resource for IT professionals seeking to optimize their log analysis workflows and stay ahead of potential issues in their systems and applications.
Check out this PowerShell Scripts link for more useful for any system administrator here at DBASco!
Leave a Reply